Liltag - A Simple Tag Manager for Your Website
I continue to work on my open-source project Econumo and at some point, I needed to add analytics scripts to the public version of the site without storing them in the repository.
One option was to use a separate template for production, but the problem is that I distribute a single build with already compiled, minified static content, making template replacement inconvenient. Another option was to use Google Tag Manager or its alternatives. However, they are more complex systems and might not be GDPR compliant, which could be an issue for some users of the open-source version.
In the end, I thought it would be simpler to create my own lightweight version of a Tag Manager that could be flexibly configured via JSON. This suits both my needs and the open-source version.
Hence, Liltag was born.
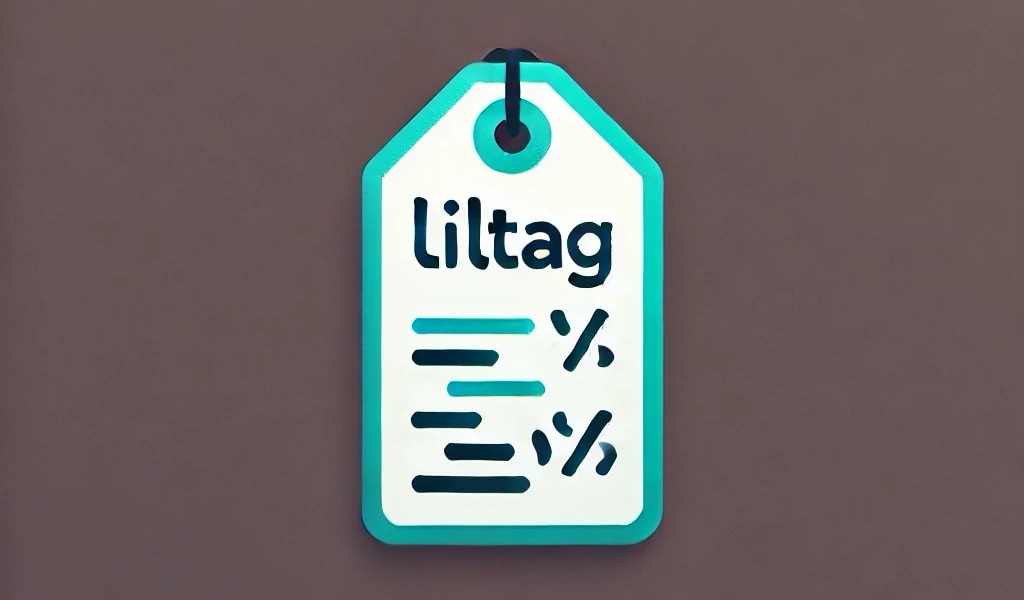
Getting it Done in 20 Minutes
The realization that I needed such a tool hit me on my way from the office to the train. The ride from downtown to home takes about 20 minutes. Considering I only had my phone with me, programming seemed problematic.
However, I thought, why not ask for help from ChatGPT? After a few prompts, I received code, repository content, and documentation. Magic!
The first version of the library was ready during the first journey and even started working by the time I got home. Over the next few days, ChatGPT and I brainstormed and tweaked the library: improved the API, did some minor refactoring, set up automatic builds on GitHub Actions, and updated the documentation.
After roughly three train rides and final testing, I released the library as open-source.
The hardest part was deciding on the feature set, as I wanted to create a simple, yet flexible solution. And I think I succeeded. As for the quality of the resulting code—there’s room for improvement, but it is already at a commendable level.
What Liltag Can Do
- Can load scripts, styles, and HTML components onto your site similarly to Google Tag Manager.
- Allows specifying in the configuration where on the page the tag will be inserted (head, body top, or body bottom).
- The tag is initialized in response to the selected event (more on this below).
Installing Liltag
Simple Liltag Integration
<script src="//deeravenger.github.io/liltag/dist/liltag.min.js" defer></script>
<script>
const lilTag = new LilTag("path_or_url/to/liltag_config.json");
lilTag.init();
</script>
You can download liltag.min.js and connect it locally.
Asynchronous Liltag Integration
<script>
(function () {
const script = document.createElement("script");
script.src = "//deeravenger.github.io/liltag/dist/liltag.min.js";
script.async = true;
script.onload = function () {
const lilTag = new LilTag("path_or_url/to/liltag_config.json");
lilTag.init();
};
document.head.appendChild(script);
})();
</script>
Configuring Liltag
Loading Configuration from URL
When installing Liltag, you can specify a URL for the configuration, which will be loaded and used to initialize the tags.
<script src="//deeravenger.github.io/liltag/dist/liltag.min.js" defer></script>
<script>
const lilTag = new LilTag("path_or_url/to/liltag_config.json");
lilTag.init();
</script>
Configuration example:
{
"tags": [
{
"id": "analytics",
"trigger": "pageLoad",
"content": "<script type=\"text/javascript\">console.log('Analytics script loaded.');</script>",
"location": "head"
}
]
}
In-Code Configuration for Liltag
const lilTag = new LilTag({
"tags": [
{
"id": "analytics",
"trigger": "pageLoad",
"content": "<script type='text/javascript'>console.log('Analytics script loaded.');</script>",
"location": "head"
}
]
});
lilTag.init();
Configuration Caching
Liltag can cache the configuration, which helps avoid redundant requests to the server and improves performance.
const lilTag = new LilTag('path_or_url/to/liltag_config.json');
lilTag.enableCache(7200); // Enable caching with a TTL of 2 hours (7200 seconds)
lilTag.init();
Full Configuration
Each tag in the configuration can contain the following fields:
- id: Unique identifier of the tag.
- trigger: Event that will initialize the tag. Available events are:
pageLoad
: Initialized on page load.domReady
: Initialized when the DOM is ready.timeDelay
: Initialized after a delay in seconds.elementVisible
: Initialized when an element becomes visible on the page.customEvent
: Initialized on a custom event.
- content: HTML component to be inserted into the page.
- location: Position where the tag will be inserted:
head
: In the head.bodyTop
: At the top of the body.bodyBottom
: At the bottom of the body.
- delay (optional): Initialization delay in seconds. Used only if the trigger is
timeDelay
. - selector (optional): Element selector for initialization. Used only if the trigger is
elementVisible
. - eventName (optional): Event name for initialization. Used only if the trigger is
customEvent
.
Conclusion
Over the past year, ChatGPT (and other neural networks) has gotten significantly better at maintaining context and understanding what’s required of it. I gradually see how my development process is transforming, adapting to various tools. At the moment, it feels like working with a junior who can quickly search for and suggest different solutions but can’t yet independently come up with and implement something new.